#include<iostream.h>
#include<conio.h>
#include<stdio.h>
#include<string.h>
#include<process.h>
#include<fstream.h>
#include<dos.h>
#include<graphics.h>
#include "c:/tc/file1.cpp"
#include "c:/tc/file2.cpp"
char fname[]="c:/temp1.bin";
class student
{
char name[20],fees[20],rollno[20],e_mail[20];
public:
int input();
void output(int,int);
int compare(char r[]);
};
struct node
{
student info;
node *link;
};
class linklist
{
node * start;
public:
linklist()
{
start=NULL;
}
void file2list();
void list2file();
void insert(student);
void show();
void search();
void delete1();
void modify();
};
void linklist::file2list()
{
ifstream f;
f.open(fname,ios::binary);
student n;
if(f.fail())
{
cout<<"\n FILE COULD NOT BE OPENED:";
return;
}
while(1)
{
f.read((char*)&n,sizeof(n));
if(f.eof())
break;
insert(n);
}
f.close();
}
void linklist::list2file()
{
ofstream f;
f.open(fname,ios::binary);
student n;
node*p=start;
while(p!=NULL)
{
n=p->info;
f.write((char*)&n,sizeof(n));
p=p->link;
}
f.close();
}
int student::input()
{
char s[4][50];
int a;
a=showform(s,4);
if(a==0)
return 0;
else
{
strcpy(rollno,s[0]);
strcpy(name,s[1]);
strcpy(fees,s[2]);
strcpy(e_mail,s[3]);
return 1;
}
}
void student::output(int x,int y)
{
outtextxy(x,y,rollno);
outtextxy(x+100,y,name);
outtextxy(x+200,y,fees);
outtextxy(x+300,y,e_mail);
}
int student::compare(char r[10])
{
if(strcmp(rollno,r)==0)
{
return 1;
}
else
{
return 0;
}
}
void linklist::delete1()
{ char temp[20];
cout<<"Enter the roll no. whose record u want to delete:-> ";
gets(temp);
student n;
node *p,*q;
int i=0;
p=start;
q=p->link;
if(start==NULL)
{ cout<<"Linked list is empty";
getch();
return;
}
while(q!=NULL)
{ n=q->info;
if(n.compare(temp)==1)
{ i++;
p->link=q->link;
q->link=NULL;
delete q;
cout<<"\n\n Record is successfully deleted";
break;
}
p=p->link;
q=q->link;
}
if(i==0)
cout<<"\n\n\n NO RECORD FOUND";
}
void linklist::search()
{
student n;
char k[10];
cout<<"\n ENTER THE ROLL NO THAT U WANT TO SEARCH:";
cin>>k;
int z=0;
node *p=start;
while(p!=NULL)
{
n=p->info;
if(n.compare(k)==1)
{
cout<<"\n RECORD IS FOUND:";
n.output(100,80);
z++;
}
p=p->link;
}
if(z==0)
{
cout<<"\n RECORD IS NOT FOUND:";
}
}
void linklist::insert(student n)
{
node*q=new node;
q->info=n;
q->link=NULL;
if(start==NULL)
{
start=q;
}
else
{
node* p=start;
while(p->link!=NULL)
{
p=p->link;
}
p->link=q;
}
}
void linklist::show()
{
cleardevice();
student n;
node *p=start;
int a=10,b=30;
while(p!=NULL)
{
n=p->info;
n.output(a,b);
b+=30;
p=p->link;
}
}
void linklist::modify()
{
char temp[20];
cout<<"enter the roll no. whose record u want to modify:-> ";
gets(temp);
student n;
node *p,*q;
int i=0;
p=start;
q=p->link;
if(start==NULL)
{ cout<<"\n\n\n Linked list is empty";
getch();
return;
}
while(q!=NULL)
{ n=q->info;
if(n.compare(temp)==1)
{ i++;
cout<<"Privious record is:-> "<<endl;
n.output(100,80);
getch();
cleardevice();
cout<<"\n\n Now enter new record:-> ";
n.input();
q->info=n;
cout<<"\n\n\n\n\n\n\n\n\n\n Record is successfully modify";
break;
}
p=p->link;
q=q->link;
}
if(i==0)
cout<<"\n\n NO RECORD FOUND";
}
void main()
{
int d,m;
d=DETECT;
initgraph(&d,&m,"c:/tc");
student n;
char choice;
linklist l;
l.file2list();
while(1)
{
cleardevice();
choice=mmenu();
cleardevice();
setbkcolor(0);
settextstyle(0,0,0);
switch (choice)
{
case 1:
if(n.input()==1)
{
l.insert(n);
l.list2file();
}
break;
case 2:
l.show();
break;
case 3:
l.search();
break;
case 4:
l.delete1();
l.list2file();
break;
case 5:
l.modify();
l.list2file();
break;
case 6:
exit(0);
}
getch();
}
}
?///////////////////////////////////////////////////////////////////////////////////
//////////////////////////file1////////////////////////////////////////////////////
#include<iostream.h>
#include<conio.h>
#include<stdio.h>
#include<graphics.h>
#include<dos.h>
#include<process.h>
int color;
void arrow(int xx,int yy,int color)
{ setcolor(color);
int a[]={xx-50,yy+7,xx-40,yy+7,xx-20,yy+20,xx-40,yy+33,xx-50,yy+33,xx-50,yy+26,xx-40,yy+26,xx-27,yy+20,xx-40,yy+14,xx-50,yy+14,xx-50,yy+7};
drawpoly(11,a);
setfillstyle(1,color);
floodfill(xx-40,yy+10,color);
}
void msetfocus(int l,int r,int t,int b,char s[])
{ arrow(l,t,8);
setcolor(15);
rectangle(l,t,r,b);
setfillstyle(1,15);
floodfill(l+2,t+2,15);
setcolor(6);
outtextxy(l+5,t,s);
}
void mlostfocus(int l,int r,int t,int b,char s[])
{ arrow(l,t,7);
setcolor(7);
rectangle(l,t,r,b);
setfillstyle(1,7);
floodfill(l+2,t+2,7);
setcolor(6);
outtextxy(l+5,t,s);
}
void men(char x[][50],int msize,int hl)
{
int lf,tp,rh,bt;
lf=175;
rh=lf+225;
tp=150;
bt=tp+40;
setcolor(5);
settextstyle(1,HORIZ_DIR,4);
outtextxy(140,60,x[0]);
settextstyle(1,HORIZ_DIR,2);
for(int i=1;i<=msize;i++)
{ if(i==hl)
msetfocus(lf,rh,tp,bt,x[i]);
else
mlostfocus(lf,rh,tp,bt,x[i]);
tp+=40;
bt+=40;
}
}
void getkey(int &j,int &k,int &l)
{ REGS n,m;
m.h.ah=0X00;
int86(22,&m,&n);
j=n.h.ah;
k=n.h.al;
m.h.ah=0X02;
int86(22,&m,&n);
l=n.h.al;
}
int mmenu()
{
settextstyle(1,HORIZ_DIR,2);
setbkcolor(7);
int ac,sc,st,z=1;
char mstr[][50]={"STUDEMT'S DATABASE","INSERT NEW RECORD ","SHOW ALL RECORD","SEARCH A RECORD","DELETE A RECORD ","MODIFY A RECORD","EXIT"};
while(1)
{
men(mstr,6,z);
getkey(sc,ac,st);
/*sound(7);
delay(100);
nosound(); */
if(sc==72 && z>=1)
{
if(z==1)
{ z=6;
continue;
}
z--;
}
else if(sc==80&& z<=6)
{
z++;
if(z==7)
z=1;
}
else if(sc==1)
return 0;
else if(sc==28)
{ return z;
}
}
getch();
}
//////////////////////////////////////////////////////////////////////////
/////////////////////////file2///////////////////////////////////////////
void setfocus(int,int,int,int,char*);
void lostfocus(int,int,int,int,char*);
void lostfocus1(int,int,int,int,char*);
int showform(char str[][50],int n)
{
cleardevice();
int z;
int cur=0,max=0;
int lf=200,rh=320,tp=20,bt=40;
char s1[2],s2[50];
int s,a,p;
strcpy(s2,"");
outtextxy(50,28,"ROLL NO.->");
outtextxy(50,78,"NAME ->");
outtextxy(50,128,"FEE ->");
outtextxy(50,178,"E_MAIL ->");
setfocus(lf,tp,rh,bt,s2);
for(int i=0;i<n;i++)
strcpy(str[i],"");
while(1)
{
getkey(s,a,p);
z=p&4;
if(s==1)
return 0;
else if(z==4 && s==31 )
return 1;
// up arrow key
else if(s==72)
{
if(cur>0)
{
lostfocus1(lf,tp,rh,bt,str[cur]);
lostfocus(lf,tp,rh,bt,str[cur]);
cur--;
strcpy(s2,str[cur]);
tp=tp-50;
bt=tp+20;
}
}
// down arrow key
else if(s==80)
{
if(cur<=max-1)
{
lostfocus1(lf,tp,rh,bt,str[cur]);
lostfocus(lf,tp,rh,bt,str[cur]);
cur++;
strcpy(s2,str[cur]);
tp=tp+50;
bt=tp+20;
}
}
// enter key
else if(a==13)
{
if(strlen(s2)!=0)
{
strcpy(str[cur],s2);
if(max<n-1)
{
max++;
}
if(cur<=max-1)
{
cur++;
lostfocus(lf,tp,rh,bt,s2);
if(cur==max&&max<n-1)
strcpy(s2,"");
else
strcpy(s2,str[cur]);
tp=tp+50;
bt=tp+20;
}
}
}
else
{
if(a==8)
{
//code for backspace key
if(strlen(s2)>0)
s2[strlen(s2)-1]='\0';
}
else
{
// code for any other alphabet
s1[0]=a;
s1[1]='\0';
strcat(s2,s1);
}
}
setfocus(lf,tp,rh,bt,s2);
}
}
// Function to write the string in the text box
void setfocus(int a,int b,int c,int d,char *f)
{
setcolor(GREEN);
rectangle(a,b,c,d);
setfillstyle(SOLID_FILL,BLACK);
floodfill(a+2,b+2,GREEN);
setcolor(YELLOW);
outtextxy(a+3,b+3,f);
}
// Function to wash the rectangle in black
void lostfocus(int a,int b,int c,int d,char *f)
{
setcolor(BLACK);
rectangle(a,b,c,d);
setfillstyle(SOLID_FILL,BLACK);
floodfill(a+2,b+2,BLACK);
setcolor(WHITE);
outtextxy(a+3,b+3,f);
}
// Function called before lostfocus to wash the rectangle
void lostfocus1(int a,int b,int c,int d,char *f)
{
setcolor(BLUE);
rectangle(a,b,c,d);
setfillstyle(SOLID_FILL,BLACK);
floodfill(a+2,b+2,BLUE);
setcolor(WHITE);
outtextxy(a+3,b+3,f);
}
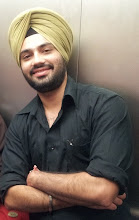
- Upinder Singh Dhami
- Bangalore, Karnataka, India
- Extending one hand to help someone has more value rather than joining two hands for prayer
Archives
-
▼
2009
(135)
-
▼
September
(132)
- program to print a random number
- program to remove the first and last occurance of ...
- program of selection sort
- program to reverse a string without using inbuilt ...
- program which show the basics of pointer
- program to count characters, words and lines in th...
- program to count vovels,digits,spaces,consonent an...
- program to sort a string
- program to find the number of substrings in a string
- program of password, where you write your password...
- program of dynamic merge
- program of dynamic sort
- program to toogle a string
- program which convert a float value to the string
- program to show the use of gotoxy
- program of merge sort
- calculate the occurance of a word in the string
- program to find the transpose of a matrix
- program to find the largest number in each row of ...
- program to find the sum of rows of the matrix
- program to find the sum of diagonal elements of th...
- program to sort the array using function
- program of seletion sort
- program to search a number present in number of ti...
- program to find the product of matrix
- program to insert the element in the array
- program for binary search of elements which are al...
- program to show basics of linklist
- program to count number of nodes in the link list
- program to count odd values of node in linklist
- program to search a node by value
- program to search a node by value and place a node...
- program to search a node by value and remove it
- program which domonstrate the use of tree
- program to remove alternate nodesin the link list
- program to reversea link list
- program to search a node by value and replace it w...
- program to remove duplicate nodes from the link list
- program to enter the name and email of students us...
- program to show inserting and deleting from a queue
- program to remove a duplicate node from link list
- program to insert an element in the queue
- program to calculate the roots of fourth order alz...
- program to solve three variable linear equation
- simple program of railway reservation
- program to replace the substring with another subs...
- program to reverse each word of the string
- program to save the output as a bitmap image
- program of sparse matrix
- program to add, sub, mul ,and divide two complex n...
- program to find the area and circumference of circ...
- program to show the basics of inline function in oops
- program to print rectangle without using inbuilt f...
- program to print rectangular bowl without using in...
- program to print 'c graphic' in the lines without ...
- program to print menu without using inbuilt functions
- program of loading
- program of loading 2
- program of moving boundries
- program to print all the ascii values
- program to print a barcode
- program to print a line
- program to print a welcome screen?
- program to demonstrate setfillstyle
- program to select the different background colours
- program which demonstrate the text style
- program to demonstrate line style
- program to demonstrate the size of text
- program to print a baby
- program of loading 1
- program of loading 2
- program to print mickey mouse
- program to print a variable box
- program to print database using moving lights arou...
- program to print menu base in graphics
- program to print menu in very good graphic form
- program to enter the values in student database
- program to enter the values of data of form in box...
- program of main menu
- program which define the pixel of the cursor while...
- program to draw lines on the screen with the help ...
- program of moving 3dimensional rectangle box
- program to show menu with the cursor
- program which takes a number from keyboard and giv...
- program which draw rectangles on the screen with t...
- program of digital switches
- program to show the basics os screen saver
- program of text box
- project of editor(like notepad) in c++
- project of banking
- project of hostel management
- project of three dimensional calculator working wi...
- graphical project of students database
- project of shooting game
- Program to find the factorial of a large number
- Solved Placement papers of different IT companies
- there is a matrix N x N .Its elements consist of e...
- program to convert binary number into decimal numb...
- A string of charater is given.Find the highest occ...
- Two sentences are given and we were required to pr...
-
▼
September
(132)
graphical project of students database
Tuesday, September 1, 2009Posted by Upinder Singh Dhami at Tuesday, September 01, 2009
Labels: projects
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment