#include<iostream.h>
#include<dos.h>
#include<graphics.h>
#include<conio.h>
#include<string.h>
#include<stdio.h>
#include<math.h>
#include<iomanip.h>
#include<process.h>
#include<fstream.h>
void password();
void add();
void display();
void update();
void del();
void search();
void exit();
struct time t;
struct date d;
fstream fin,fout,fio;
void ctd()
{
gettime(&t); gotoxy(45,50);
printf("Current Time :%2d:%02d:%02d.%02d\n",
t.ti_hour,t.ti_min,t.ti_sec,t.ti_hund);
getdate(&d);gotoxy(45,48);
printf("Current Date:%d-%d-%d\n",d.da_day,d.da_mon,d.da_year);
}
class student
{
private:
int stid;
char name[20];
char sex[10];
char course[20];
char trade[20];
int semester;
int fee;
char fpaid[10];
int rn,bc;
char rt[12];
public:
void getnewdata()
{
cout<<"enter new semester";
cin>>semester;
cout<<"enter new room no";
cin>>rn;
}
int putstid()
{
return(stid);
}
void getdata()
{ gotoxy(30,14);
cout<<"ENTER STUDENT ID :";gotoxy(30,16);
cin>>stid;
cout<<"ENTER NAME :";gotoxy(30,18);
cin>>name;
cout<<"ENTER THE ROOM ALLOTED :";gotoxy(30,20);
cin>>rn;
cout<<"ENTER SEX :";gotoxy(30,22);
cin>>sex;
cout<<"ENTER COURSE :";gotoxy(30,24);
cin>>course;
cout<<"ENTER TRADE :";gotoxy(30,26);
cin>>trade;
cout<<"ENTER SEMESTER";gotoxy(30,28);
cin>>semester;
cout<<"ENTER BATCH";gotoxy(30,30);
cin>>bc;
}
void putrt(char* p)
{
strcpy(rt,p);
}
void putdata()
{ gotoxy(30,14);
cout<<"STUDENT ID IS"<<"\t"<<"STUDENTNAME"<<"\t"<<"ROOMALLOTED"<<"\t"<<"SEX"<<"\t"<<"COURSE"<<"\t"<<"TRADE"<<"\t"<<"SEMSETER"<<"\t"<<"BATCH"<<endl;
cout<<name<<"\t"<<rn<<"\t"<<sex<<"\t"<<course<<"\t"<<trade<<"\t"<<semester<<"\t"<<bc<<endl;
}
}s1;
class personal
{
private:
int rollno;
char name[20];
char sex[10];
char bg[20];
char dob[20];
char fn[20];
char mn[20];
char adr[50];
long phn;
int rn;
public:
void getnewaddress()
{
cout<<"ENTER NEW ADDRESS";
cin>>adr;
}
char * getname()
{
return(name);
}
int getrollno()
{
return(rollno);
}
void getdata()
{
gotoxy(25,18);
textcolor(23);
cout<<" ENTER STUDENT ID :";gotoxy(25,20);
cin>>rollno;
cout<<"ENTER NAME :";gotoxy(25,22);
cin>>name;
cout<<"ENTER SEX :";gotoxy(25,24);
cin>>sex;
cout<<"ENTER BLOOD GROUP :";gotoxy(25,26);
cin>>bg;
cout<<"ENTER DATE OF BIRTH :"; gotoxy(25,28);
cin>>dob;
cout<<"ENTER FATHER NAME :"; gotoxy(25,30);
cin>>fn;
cout<<"ENTER MOTHER NAME :";gotoxy(25,32);
cin>>mn;
cout<<"ENTER THE ADDRESS :";gotoxy(25,34);
cin>>adr;
cout<<"ENTER PHONE NO. :" ;gotoxy(25,36);
cin>>phn;
}
void putdata()
{
cout<<"SID"<<"\t"<<"NAME"<<"\t"<<"SEX"<<"\t"<<"BGROUP"<<"\t"<<"FATHERNAME"<<"\t"<<"MOTHERNAME"<<"\t"<<"ADDRESS"<<"\t"<<"PNO."<<endl;
cout<<rollno<<"\t "<<name<<"\t"<<sex<<"\t"<<bg<<"\t " <<fn<<"\t "<<mn<<"\t "<<adr<<"\t "<<phn<<"\t "<<rn<<endl;
}
}p1 ;
class room
{
private:
int rn, fan,tubelight,ac,cooler,tc,bed,cupboard;
char rt[12];
char avail;
public:
int putrn()
{
return(rn);
}
void grn(int p)
{
rn=p;
}
void getrn()
{
cout<<"ENTER NEW ROOM NUMBER :";
cin>>rn;
}
void getdata()
{
gotoxy(25,25);
textcolor(13);
/* cout<<"ENTER ROOM NUMBER : ";cout<<endl;
cin>>rn; */
cout<<"ENTER NUMBER OF FAN AVAILABLE :";cout<<endl;
cin>>fan;
cout<<"ENTER NUMBER OF TUBELIGHT AVAIABLE : ";cout<<endl;
cin>>tubelight;
cout<<"ENTER NUMBER OF AC AVAILABLE : ";cout<<endl;
cin>>ac;
cout<<"ENTER NUMBER OF COOLER AVAILABLE :";cout<<endl;
cin>>cooler;
cout<<"ENTER NUMBER OF CUPBOARD AVAILABLE :";cout<<endl;
cin>>cupboard;
cout<<"ENTER NUMBER OF TABLE CHAIR AVAILABLE :";cout<<endl;
cin>>tc;
cout<<"ENTER THE NUMBER OF BED : ";cout<<endl;
cin>>bed;
cout<<"ENTER NUMBER OF ROOM AVAILABLE :";cout<<endl;
cin>>avail;
cout<<"ENTER ROOM TYPE";cout<<endl;
cout<<"1. SINGLET ROOM :"<<endl;
cout<<"2. DOUBLET ROOM :"<<endl;
cout<<"3. TRIPLET ROOM :"<<endl;
cout<<"PLEASE ENTERED CHOICE :"<<endl;
int choice;
cin>>choice;
if(choice==1)
strcpy(rt,"singlet");
else if(choice==2)
strcpy(rt,"doublet");
else strcpy(rt,"triplet");
}
void putdata()
{
cout<<"ROOMNO"<<"\t"<<"FAN"<<"\t"<<"TUBE"<<"\t"<<"AC"<<"\t"<<"COOLER"<<"\t"<<"CUPBOARD"<<"\t"<<"TABLE"<<"\t"<<"BED"<<"\t"<<"ROOM"<<endl;
cout<<rn<<"\t "<<fan<<"\t "<<tubelight<<"\t"<<ac<<"\t"<<cooler<<"\t "<<cupboard<<"\t " <<tc<<"\t "<<bed<<"\t "<<avail<<endl;
}
}r1,r2;
void main()
{
// password();
int gmode,gdriver=DETECT;
initgraph(&gdriver,&gmode,"l:\tc\bgi");
char ch;
do
{
clrscr();
ctd();
gotoxy(25,14); sound(600);
delay(500);
nosound();
cout<<"======================================"; gotoxy(30,14);
cout<<"*MAIN MENU*"<<setw(20)<<endl;gotoxy(24,16);
cout<<" 1. ADD THE RECORD:"<<endl;gotoxy(25,18);
cout<<" 2. DISPLAY THE RECORD:"<<endl;gotoxy(25,20);
cout<<" 3. SEARCH THE RECORD:"<<endl;gotoxy(25,22);
cout<<" 4. UPDATE RECORD:"<<endl;gotoxy(25,24);
cout<<" 5. DELETE RECORD:"<<endl;gotoxy(25,26);
cout<<" 6. EXIT"<<endl;gotoxy(25,28);
cout<<"PLEASE ENTER THE CHOICE :"; gotoxy(25,30);
cout<<"=======================================";
cin>>ch;
switch(ch) //main switch
{
case '1': add();break;
case '2': display();break;
case '3': search();break;
case '4': update();break;
case '5': del(); break;
case '6': exit();
}
}while(ch!='6');
closegraph();
getch();
}
// end of main function
void password()
{ ctd();
gotoxy(15,20);
textcolor(6);
textbackground(750);
cout<< " *******************************************************"<<endl;gotoxy(15,20);
cout<< " *******************************************************"<<endl;gotoxy(15,21);
cout<< " *******************************************************"<<endl;gotoxy(15,22);
cout<< " ********** PROJECT **********"<<endl;gotoxy(15,23);
cout<< " ********** ON **********"<<endl;gotoxy(15,24);
cout<< " ********** HOSTEL MANAGEMENT **********"<<endl;gotoxy(15,25);
cout<< " ********** **********"<<endl;gotoxy(15,26);
cout<< " ********** MADE BY: **********"<<endl;gotoxy(15,27);
cout<< " ********** VAMA VARUN **********"<<endl;gotoxy(15,28);
cout<< " ********** VISHAL VISHAL **********"<<endl;gotoxy(15,29);
cout<< " *******************************************************"<<endl;gotoxy(15,30);
cout<< " *******************************************************"<<endl;gotoxy(15,31);
cout<< " *******************************************************"<<endl;gotoxy(15,32);
getch();
// password code
char un[20]="vishal",pw[20]="manish",un1[20],pw1[20];
for(int i=0;i<3;i++) //for loop
{
int j=0;
textcolor(6);
textbackground(700);
clrscr();
ctd();
gotoxy(26,26);
cout<<"PLEASE ENTER USER NAME : ";
gotoxy(50,26);
cin>>un1;
clrscr();
ctd();
gotoxy(26,26);
textcolor(6);
textbackground(700);
cout<<"PLEASE ENTER YOUR PASSWORD >%>%>%>%>%> ";
char ch; j=0;
do
{
ch=getch();
pw1[j]=ch;
if(ch=='\n'||ch=='\t'||ch=='\r')
break;
else
{
cout<<"*";
j++;
}
}while(ch!='\r');
pw1[j]='\0';
gotoxy(25,15);
if(strcmp(un,un1)==0 && strcmp(pw,pw1)==0)
{
clrscr();
ctd(); //right password
gotoxy(30,12);
textcolor(6);
textbackground(700);
cout<<"PASSWORD................IS....CONFERMED";
getch();
clrscr();
gotoxy(30,12);
cout<<" WELCOME ";gotoxy(30,14);
cout<<" TO ";gotoxy(30,16);
cout<<" HOSTEL ";
getch();
break;
}
else
{
if(i==2) //wrong code
exit(0);
} clrscr();
gotoxy(30,14);
cout<<" WRONG......... PASSWORD.........";
getch();
clrscr();
gotoxy(30,14);
cout<<"TRY AGAIN.......................";
getch();
}
} //end of password function
void add()
{
char choice;
do
{
clrscr();
ctd();
gotoxy(29,14);
cout<<"*ADD RECORD*"<<endl;
gotoxy(25,18);
cout<<"a.ROOM RECORD"<<endl; gotoxy(25,20);
cout<<"b.STUDENT RECORD ::PERSONAL INFORMATION"<<endl; gotoxy(25,22);
cout<<"c.STUDENT RECORD ::STUDENT ID "<<endl; gotoxy(25,24);
cout<<"d.TO EXIT";
cout<<"ENTER CHOICE ";
cin>>choice;
clrscr();
switch(choice)
{
case 'a':
cout<<"STUDENT ID"<<endl;gotoxy(30,14);
char a;
clrscr();
ctd();
gotoxy(25,25);
cout<<" ROOM INFORMATION : "<<endl;gotoxy(25,26);
cout<<"1. SINGLET ROOM :"<<endl;gotoxy(25,27);
cout<<"2. DOUBLET ROOM :"<<endl; gotoxy(25,28);
cout<<"3. TRIPLET ROOM :"<<endl; gotoxy(25,29);
cout<<"PLEASE ENTERED CHOICE :"<<endl;
cin>>a;
switch(a)
{
case '1':
cout<<"*SINGLET ROOM*" <<endl;
cout<<"SINGLET"<<endl;
cout<<"CHARGES ARE AS FOLLOWS:"<<endl;
cout<<"ROOM CHARGES ARE:Rs 1500 PER MONTH (AC)"<<endl;
getch();
break;
case '2':
cout<<" *DOUBLET ROOM*" <<endl;
cout<<"DOUBLET "<<endl;
cout<<"CHARGES ARE AS FOLLOWS:"<<endl;
cout<<"ROOM CHARGES ARE:Rs 1200 PER MONTH (AC)"<<endl;
getch();
break;
case '3':
cout<<" *TRIPLET ROOM*"<<endl;
cout<<"TRIPLET "<<endl;
cout<<"CHARGES ARE AS FOLLOWS:"<<endl;
cout<<"ROOM CHARGES ARE:Rs 1000 PER MONTH (AC)"<<endl;
getch();
break;
default :
{
cout<<" *ENTER APPOPRIATE CHOICE*"<<endl;
cout<<"WRONG CHOICE "<<endl;
getch();
}
}
cout<<"ROOM RECORD :"<<endl; gotoxy(10,11);
int flag=0;
clrscr();
ctd();
cout<<"ENTER ROOM INFORMATION"<<endl;
fio.open("rm.dat",ios::out|ios::in);
fio.seekg(0,ios::beg);
if(!fio)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
getch();
}
else
{
int rn;
cout<<"enter room no";
cin>>rn;
fio.seekg(0,ios::beg);
while(fio.read((char*)&r1,sizeof(r1)))
{
getch();
if(rn==r1.putrn())
{
flag=1;
break;
}
}
fio.clear();
fio.close();
if(flag==1)
{
cout<<"room already exist";
getch();
return;
}
r2.getdata();
r2.grn(rn);
fout.open("rm.dat",ios::out|ios::ate);
cout<<"WANT TO SAVE THE DATA? PRESS Y/N.";
cin>>choice;
if(choice=='y')
{
fout.write( (char *)&r2,sizeof(r2));
fout.clear();
cout<<"record saved";
getch();
}
fout.close();
}
break;
case 'c':
cout<<"STUDENT RECORD:STUDENT ID "<<endl; gotoxy(10,11);
int flag1=0;
clrscr();
ctd();
cout<<"ENTER STUDENT ID"<<endl;
fio.open("st.dat",ios::out|ios::in);
fio.seekg(0,ios::beg);
if(!fio)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
getch();
}
else
{
int rn5;
cout<<"enter student id";
cin>>rn5;
fio.seekg(0,ios::beg);
while(fio.read((char*)&s1,sizeof(s1)))
{
getch();
if(rn5==s1.putstid())
{
flag1=1;
break;
}
}
fio.clear();
fio.close();
if(flag1==1)
{
cout<<"id already exist";
getch();
return;
}
s1.getdata();
s1.putstid();
fout.open("st.dat",ios::out|ios::ate);
cout<<"WANT TO SAVE THE DATA? PRESS Y/N.";
cin>>choice;
if(choice=='y')
{
fout.write( (char *)&s1,sizeof(s1));
fout.clear();
cout<<"record saved";
getch();
}
fout.close();
}
break;
/* clrscr();
ctd();
fout.open("pi.dat",ios::app);
if(!fout)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
}
else
{
clrscr();
cout<<"ENTER THE DATA :"<<endl;
p1.getdata();
cout<<"WANT TO BE SAVE THEN PRESS Y:";
cin>>choice;
if(choice=='y')
{
fout.write((char*)&p1,sizeof(p1));
fout.clear();
getch();
}
fout.close();
}
break; */
case 'b':
cout<<"STUDENT RECORD:PERSONAL INFORMATION "<<endl; gotoxy(10,11);
int flag2=0;
clrscr();
ctd();
cout<<"ENTER PERSONAL INFORMATION"<<endl;
fio.open("pi.dat",ios::out|ios::in);
fio.seekg(0,ios::beg);
if(!fio)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
getch();
}
else
{
char* p;
char name2[15];
cout<<"enter student name";
cin>>name2;
fio.seekg(0,ios::beg);
while(fio.read((char*)&p1,sizeof(p1)))
{
getch();
if(strcmp(name2,p)==0)
{
flag2=1;
break;
}
}
fio.clear();
fio.close();
if(flag2==1)
{
cout<<"name already exist";
getch();
return;
}
p1.getdata();
// p1.get(name2);
fout.open("pi.dat",ios::out|ios::ate);
cout<<"WANT TO SAVE THE DATA? PRESS Y/N.";
cin>>choice;
if(choice=='y')
{
fout.write( (char *)&p1,sizeof(p1));
fout.clear();
cout<<"record saved";
getch();
}
fout.close();
}
break;
/* clrscr();
ctd();
gotoxy(30,14);
cout<<"PERSONAL INFORMATION"<<endl;
fout.open("pi.dat",ios::app);
if(!fout)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
}
else
{
cout<<"ENTER THE DATA :"<<endl;
p1.getdata();
cout<<"want to save then press y";
cin>>choice;
if(choice=='y')
{
fout.write( (char *)&p1,sizeof(p1));
fout.clear();
getch();
}
fout.close();
} */
// main add switch case
}
}while(choice!='d');
getch();
}
void display()
{ char choice;
do
{ clrscr(); //label for add menu
ctd();
gotoxy(29,14);
cout<<"*DISPLAY RECORD*"<<endl;
gotoxy(25,18);
cout<<"a.ROOM RECORD "<<endl; gotoxy(25,20);
cout<<"b.STUDENT RECORD ::PERSONAL INFORMATION "<<endl; gotoxy(25,22);
cout<<"c.STUDENT RECORD ::STUDENT ID"<<endl;gotoxy(25,24);
cout<<"d.BACK TO MAIN MENU"<<endl;gotoxy(25,26);
cout<<"ENTER CHOICE ";
cin>>choice;
clrscr();
ctd();
switch(choice) // case 2a student record
{
case 'a':cout<<"ROOM RECORD"<<endl; gotoxy(10,11);
cout<<"ROOM INFORMATION"<<endl;
fin.open("rm.dat",ios::in);
fin.seekg(0,ios::beg);
if(!fin)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
getch();
}
else
{
clrscr();
while(fin.read((char *)&r1,sizeof(r1)))
{
r1.putdata();
fin.clear();
getch();
}
fin.close();
}
break;
case 'b':clrscr();
ctd();
gotoxy(30,14);
cout<<"STUDENT RECORD :"<<endl; gotoxy(25,18);
cout<<"PERSONAL INFORMATION"<<endl;
fin.open("pi.dat",ios::in);
if(!fin)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
getch();
}
else
{
while(fin.read((char *)&p1,sizeof(p1)))
{
p1.putdata();
cout<<endl;
fin.clear();
getch();
}
fin.close();
}
break;
case 'c': gotoxy(30,14);
cout<<"STUDENT INFORMATION"<<endl;
cout<<"STUDENT ID"<<endl;
fin.open("st.dat",ios::in);
if(!fin)
{
cout<<"FILE CAN'T BE OPENED"<<endl;
}
else
{
while(fin.read((char*)&s1,sizeof(s1)))
{
s1.putdata();
fin.clear();
getch();
}
fin.close();
}
break;
case 'd': cout<<"back to main menu";
getch();
}
}while(choice!='d');
getch();
}
void search()
{
clrscr();
ctd();
char choice;
do
{
clrscr();
ctd();
gotoxy(29,14);
textcolor(25);
textbackground(373);
cout<<"*SEARCH RECORD*"<<endl;
char choice22; gotoxy(25,18);
cout<<"a.STUDENT RECORD BY NAME "<<endl; gotoxy(25,20);
cout<<"b.student id "<<endl; gotoxy(25,22);
cout<<"c.room no "<<endl; gotoxy(25,24);
cout<<"d. back to main menu "<<endl; gotoxy(25,26);
cout<<"ENTER CHOICE ";
cin>>choice;
clrscr();
ctd();
switch(choice)
{
case 'a' : gotoxy(30,14);
cout<<"STUDENT RECORD BY NAME"<<endl;
char *p; char name[15];int flag=0;
cout<<"ENTER STUDENT NAME";
cin>>name;
fin.open("pi.dat",ios::in);
while(fin.read((char*)&p1,sizeof(p1)))
{
p=p1.getname();
if(strcmp(name,p)==0)
{
p1.putdata();
getch();
flag=1;
}
}
if(flag==0)
{
cout<<"RECORD DOES NOT EXIST";
getch();
}
fin.close(); //search main switch
break;
case 'b' : gotoxy(30,14);
int roll;
cout<<"STUDENT RECORD BY ID"<<endl;
cout<<"ENTER THE STUDENT ID";
cin>>roll;
flag=0;
fin.open("st.dat",ios::in);
while(fin.read((char*)&s1,sizeof(s1)))
{
if(roll==s1.putstid())
{
s1.putdata();
getch();
flag=1;
}
}
if(flag==0)
{
cout<<"RECORD DOES NOT EXIST";
getch();
}
fin.close();
break;
case 'c': clrscr();
gotoxy(30,16);
cout<<"ROOM INFORMATION "<<endl;
int rn;
flag=0;
cout<<"ENTER ROOM NUMBER ";
cin>>rn;
fin.open("rm.dat",ios::in);
while(fin.read((char*)&r1,sizeof(r1)))
{
if(rn==r1.putrn())
{
r1.putdata();
flag=1;
getch();
}
fin.clear();
}
if(flag==0)
{
cout<<"RECORD DOES NOT EXIST"<<endl;
getch();
}
fin.close();
break;
case 'd': cout<<"BACK TO MAIN MENU";
getch(); }
} while(choice!='d'); //main serch
getch();
}
void update()
{
clrscr();
cout<<"update"<<endl;
char choice;
do
{
clrscr();
gotoxy(29,14);
textcolor(25);
textbackground(373);
cout<<"*UPDATE RECORD*"<<endl;
char choice22; gotoxy(25,18);
cout<<"a.ROOM RECORD"<<endl; gotoxy(25,20);
cout<<"b.STUDENT RECORD:: PERSONAL INFORMATION "<<endl; gotoxy(25,22);
cout<<"c.STUDENT RECORD::STUDENT ID "<<endl; gotoxy(25,24);
cout<<"d. BACK TO MAIN MENU "<<endl; gotoxy(25,26);
cout<<"ENTER CHOICE ";
cin>>choice;
clrscr();
int flag;
switch(choice)
{
case 'a': clrscr();
gotoxy(30,16);
cout<<"ROOM INFORMATION "<<endl;
int rn;
flag=0;
cout<<"ENTER ROOM NO";
cin>>rn;
fin.open("rm.dat",ios::in|ios::out|ios::ate);
fin.seekg(0,ios::beg);
if(!fin)
{
cout<<"FILE CAN NOT OPENED";
getch(); return;
}
else
{
while(fin.read((char*)&r1,sizeof(r1)))
{ cout<<"rn"<<r1.putrn()<<endl;
if(rn==r1.putrn())
{
int p=fin.tellp();
fin.seekp(p-sizeof(r1));
r1.getrn();
fin.write((char*)&r1,sizeof(r1));
fin.clear();
flag=1;
getch();
break;
}
} fin.close();
if(flag==0)
{
cout<<"RECORD DOES NOT EXIST"<<endl;
return;
}
cout<<"RECORD IS UPDATED"<<endl;
getch();
}
break;
case 'b' : gotoxy(30,14);
cout<<"STUDENT RECORD BY NAME"<<endl;
char *p; char name[15];int flag=0;
cout<<"ENTER STUDENT NAME";
cin>>name;
fin.open("pi.dat",ios::in|ios::ate|ios::out);
fin.seekg(0,ios::beg);
while(fin.read((char*)&p1,sizeof(p1)))
{
if(strcmp(name,p1.getname())==0)
{
int p=fin.tellp();
p1.getnewaddress();
fin.seekp(p-sizeof(p1));
fin.write((char*)&p1,sizeof(p1));
fin.clear();
p1.putdata();
getch();
flag=1;
}
}
if(flag==0)
{
cout<<"RECORD DOES NOT EXIST";
getch();
}
fin.close();
cout<<"RECORD IS UPDATED"<<endl;
getch(); //update main switch
break;
case 'c' : gotoxy(30,14);
int roll;
cout<<"STUDENT RECORD BY STUDENT ID"<<endl;
cout<<"ENTER THE STUDENT ID";
cin>>roll;
flag=0;
fin.open("st.dat",ios::in|ios::ate|ios::out);
fin.seekg(0,ios::beg);
while(fin.read((char*)&s1,sizeof(s1)))
{
if(roll==s1.putstid())
{
int p=fin.tellp();
s1.getnewdata();
fin.seekp(p-sizeof(s1));
fin.write((char*)&s1,sizeof(s1));
fin.clear();
getch();
flag=1;
}
}
if(flag==0)
{
cout<<"RECORD DOES NOT EXIST";
getch();
}
cout<<"RECORD IS UPDATED";
getch();
fin.close();
break;
case 'd': cout<<"back to main menu";
return;
}
} while(choice!='d'); //main update.
getch();
}
void del()
{ clrscr();
cout<<"delete"<<endl;
char choice;
do
{
clrscr();
gotoxy(29,14);
textcolor(25);
textbackground(373);
cout<<"*DELETE RECORD*"<<endl;
char choice22; gotoxy(25,18);
cout<<"a.DELETE THROUGH ROOM NUMBER"<<endl; gotoxy(25,20);
cout<<"b.DELETE THROUGH SYUDENT ID "<<endl; gotoxy(25,22);
cout<<"c.DELETE THROUGH STUDENT NAME "<<endl; gotoxy(25,24);
cout<<"d. BACK TO MAIN MENU "<<endl; gotoxy(25,26);
cout<<"ENTER CHOICE ";
cin>>choice;
clrscr();
int flag;
switch(choice)
{
case 'a': clrscr();
gotoxy(30,16);
cout<<"DELETE THROUGH ROOM NO "<<endl;
int rn;
flag=0;
cout<<"ENTER ROOM NO";
cin>>rn;
fout.open("temp.dat",ios::in|ios::out|ios::app);
fout.seekg(0, ios::beg);
fin.open("rm.dat",ios::in|ios::out|ios::app);
fin.seekg(0, ios::beg);
while(fin.read((char*)&r1,sizeof(r1)))
{
if(rn==r1.putrn())
{
cout<<"RECORD DELETED";
getch();
flag=1;
}
else
{ fout.write((char*)&r1,sizeof(r1));
fout.clear();
fin.clear();
}
}
fin.close();
fout.close();
if(flag==0)
{
cout<<"RECORD NOT FOUND";
getch();
}
remove("rm.dat");
rename("temp.dat","rm.dat");
break;
case 'b':
clrscr();
gotoxy(30,16);
cout<<"DELETE THROUGH STUDENT ID "<<endl;
int rn1;
flag=0;
cout<<"ENTER STUDENT ID";
cin>>rn1;
fout.open("temp.dat",ios::in|ios::out|ios::app);
fout.seekg(0, ios::beg);
fin.open("st.dat",ios::in|ios::out|ios::app);
fin.seekg(0, ios::beg);
while(fin.read((char*)&s1,sizeof(s1)))
{
if(rn1==s1.putstid())
{
cout<<"RECORD DELETED";
getch();
flag=1;
}
else
{ fout.write((char*)&s1,sizeof(s1));
fout.clear();
fin.clear();
}
}
fin.close();
fout.close();
if(flag==0)
{
cout<<"RECORD NOT FOUND";
getch();
}
remove("st.dat");
rename("temp.dat","st.dat");
break;
case 'c':
clrscr();
gotoxy(30,16);
cout<<"DELETE THROUGH STUDENT NAME"<<endl;
char name[15];
char* p;
flag=0;
cout<<"ENTER STUDENT NAME";
cin>>name;
fout.open("temp.dat",ios::in|ios::out|ios::app);
fout.seekg(0, ios::beg);
fin.open("pi.dat",ios::in|ios::out|ios::app);
fin.seekg(0, ios::beg);
while(fin.read((char*)&p1,sizeof(p1)))
{
p=p1.getname();
if(strcmp(name,p)==0)
{
cout<<"RECORD DELETED";
getch();
flag=1;
}
else
{ fout.write((char*)&s1,sizeof(s1));
fout.clear();
fin.clear();
}
}
fin.close();
fout.close();
if(flag==0)
{
cout<<"RECORD NOT FOUND";
getch();
}
remove("pi.dat");
rename("temp.dat","pi.dat");
break;
case 'd':
cout<<"BACK TO MAIN MENU";
getch();
return;
}}while(choice!='d');
getch();
}
void exit()
{
clrscr();
gotoxy(35,25);
cout<<"BYE BYE";
getch();
exit(1);
}
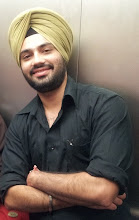
- Upinder Singh Dhami
- Bangalore, Karnataka, India
- Extending one hand to help someone has more value rather than joining two hands for prayer
Archives
-
▼
2009
(135)
-
▼
September
(132)
- program to print a random number
- program to remove the first and last occurance of ...
- program of selection sort
- program to reverse a string without using inbuilt ...
- program which show the basics of pointer
- program to count characters, words and lines in th...
- program to count vovels,digits,spaces,consonent an...
- program to sort a string
- program to find the number of substrings in a string
- program of password, where you write your password...
- program of dynamic merge
- program of dynamic sort
- program to toogle a string
- program which convert a float value to the string
- program to show the use of gotoxy
- program of merge sort
- calculate the occurance of a word in the string
- program to find the transpose of a matrix
- program to find the largest number in each row of ...
- program to find the sum of rows of the matrix
- program to find the sum of diagonal elements of th...
- program to sort the array using function
- program of seletion sort
- program to search a number present in number of ti...
- program to find the product of matrix
- program to insert the element in the array
- program for binary search of elements which are al...
- program to show basics of linklist
- program to count number of nodes in the link list
- program to count odd values of node in linklist
- program to search a node by value
- program to search a node by value and place a node...
- program to search a node by value and remove it
- program which domonstrate the use of tree
- program to remove alternate nodesin the link list
- program to reversea link list
- program to search a node by value and replace it w...
- program to remove duplicate nodes from the link list
- program to enter the name and email of students us...
- program to show inserting and deleting from a queue
- program to remove a duplicate node from link list
- program to insert an element in the queue
- program to calculate the roots of fourth order alz...
- program to solve three variable linear equation
- simple program of railway reservation
- program to replace the substring with another subs...
- program to reverse each word of the string
- program to save the output as a bitmap image
- program of sparse matrix
- program to add, sub, mul ,and divide two complex n...
- program to find the area and circumference of circ...
- program to show the basics of inline function in oops
- program to print rectangle without using inbuilt f...
- program to print rectangular bowl without using in...
- program to print 'c graphic' in the lines without ...
- program to print menu without using inbuilt functions
- program of loading
- program of loading 2
- program of moving boundries
- program to print all the ascii values
- program to print a barcode
- program to print a line
- program to print a welcome screen?
- program to demonstrate setfillstyle
- program to select the different background colours
- program which demonstrate the text style
- program to demonstrate line style
- program to demonstrate the size of text
- program to print a baby
- program of loading 1
- program of loading 2
- program to print mickey mouse
- program to print a variable box
- program to print database using moving lights arou...
- program to print menu base in graphics
- program to print menu in very good graphic form
- program to enter the values in student database
- program to enter the values of data of form in box...
- program of main menu
- program which define the pixel of the cursor while...
- program to draw lines on the screen with the help ...
- program of moving 3dimensional rectangle box
- program to show menu with the cursor
- program which takes a number from keyboard and giv...
- program which draw rectangles on the screen with t...
- program of digital switches
- program to show the basics os screen saver
- program of text box
- project of editor(like notepad) in c++
- project of banking
- project of hostel management
- project of three dimensional calculator working wi...
- graphical project of students database
- project of shooting game
- Program to find the factorial of a large number
- Solved Placement papers of different IT companies
- there is a matrix N x N .Its elements consist of e...
- program to convert binary number into decimal numb...
- A string of charater is given.Find the highest occ...
- Two sentences are given and we were required to pr...
-
▼
September
(132)
project of hostel management
Tuesday, September 1, 2009Posted by Upinder Singh Dhami at Tuesday, September 01, 2009
Labels: projects
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment