#include<iostream.h>
#include<conio.h>
#include<graphics.h>
#include<dos.h>
#include<string.h>
void getkey(int&,int&,int&);
void setfocus(int,int,int,int,char*);
void lostfocus(int,int,int,int,char*);
void lostfocus1(int,int,int,int,char*);
void main()
{
clrscr();
// Array of String
char str[3][50];
// max will give how many strings have been enterd in the array and
// cur will give the current position in the array of string
int cur=0,max=0;
// parameters of rectangle
int lf=200,rh=320,tp=20,bt=40;
//s2 will have the string being enterd by the user
char s1[2],s2[50];
int s,a,p;
// Graphics Initialisation
int d,m;
d=DETECT;
initgraph(&d,&m,"c:\\tc");
outtextxy(50,28,"ROLL NO.->");
outtextxy(50,78,"NAME ->");
outtextxy(50,128,"FEE ->");
outtextxy(50,178,"E_MAIL ->");
strcpy(s2,"");
setfocus(lf,tp,rh,bt,s2);
// COpying empty string in array of string to avoid garbage values
for(int i=0;i<3;i++)
strcpy(str[i],"");
while(1)
{
getkey(s,a,p);
//press esc key to break
if(s==1)
break;
// up arrow key
else if(s==72)
{
if(cur>0)
{
lostfocus1(lf,tp,rh,bt,str[cur]);
lostfocus(lf,tp,rh,bt,str[cur]);
cur--;
strcpy(s2,str[cur]);
tp=tp-50;
bt=tp+20;
}
}
// down arrow key
else if(s==80)
{
if(cur<max)
{
lostfocus1(lf,tp,rh,bt,str[cur]);
lostfocus(lf,tp,rh,bt,str[cur]);
cur++;
strcpy(s2,str[cur]);
tp=tp+50;
bt=tp+20;
}
}
// enter key
else if(a==13)
{
if(strlen(s2)!=0)
{
strcpy(str[cur],s2);
if(max<2)
{
max++;
}
if(cur<max)
{
cur++;
lostfocus(lf,tp,rh,bt,s2);
if(cur==max&&max<2)
strcpy(s2,"");
else
strcpy(s2,str[cur]);
tp=tp+50;
bt=tp+20;
}
}
}
else
{
if(a==8)
{
//code for backspace key
if(strlen(s2)>0)
s2[strlen(s2)-1]='\0';
}
else
{
// code for any other alphabet
s1[0]=a;
s1[1]='\0';
strcat(s2,s1);
}
}
setfocus(lf,tp,rh,bt,s2);
} //while loop closed
getch();
closegraph();
}
void getkey(int &sc,int &ac,int &sp)
{
REGS ix,ox;
ix.h.ah=0x00;
int86(0x16,&ix,&ox);
sc=ox.h.ah;
ac=ox.h.al;
ix.h.ah=0x02;
int86(0x16,&ix,&ox);
sp=ox.h.al;
}
// Function to write the string in the text box
void setfocus(int a,int b,int c,int d,char *f)
{
setcolor(GREEN);
rectangle(a,b,c,d);
setfillstyle(SOLID_FILL,BLACK);
floodfill(a+2,b+2,GREEN);
setcolor(YELLOW);
outtextxy(a+3,b+3,f);
}
// Function to wash the rectangle in black
void lostfocus(int a,int b,int c,int d,char *f)
{
setcolor(BLACK);
rectangle(a,b,c,d);
setfillstyle(SOLID_FILL,BLACK);
floodfill(a+2,b+2,BLACK);
setcolor(WHITE);
outtextxy(a+3,b+3,f);
}
// Function called before lostfocus to wash the rectangle
void lostfocus1(int a,int b,int c,int d,char *f)
{
setcolor(BLUE);
rectangle(a,b,c,d);
setfillstyle(SOLID_FILL,BLACK);
floodfill(a+2,b+2,BLUE);
setcolor(WHITE);
outtextxy(a+3,b+3,f);
}
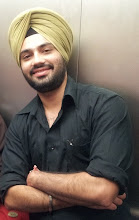
- Upinder Singh Dhami
- Bangalore, Karnataka, India
- Extending one hand to help someone has more value rather than joining two hands for prayer
Archives
-
▼
2009
(135)
-
▼
September
(132)
- program to print a random number
- program to remove the first and last occurance of ...
- program of selection sort
- program to reverse a string without using inbuilt ...
- program which show the basics of pointer
- program to count characters, words and lines in th...
- program to count vovels,digits,spaces,consonent an...
- program to sort a string
- program to find the number of substrings in a string
- program of password, where you write your password...
- program of dynamic merge
- program of dynamic sort
- program to toogle a string
- program which convert a float value to the string
- program to show the use of gotoxy
- program of merge sort
- calculate the occurance of a word in the string
- program to find the transpose of a matrix
- program to find the largest number in each row of ...
- program to find the sum of rows of the matrix
- program to find the sum of diagonal elements of th...
- program to sort the array using function
- program of seletion sort
- program to search a number present in number of ti...
- program to find the product of matrix
- program to insert the element in the array
- program for binary search of elements which are al...
- program to show basics of linklist
- program to count number of nodes in the link list
- program to count odd values of node in linklist
- program to search a node by value
- program to search a node by value and place a node...
- program to search a node by value and remove it
- program which domonstrate the use of tree
- program to remove alternate nodesin the link list
- program to reversea link list
- program to search a node by value and replace it w...
- program to remove duplicate nodes from the link list
- program to enter the name and email of students us...
- program to show inserting and deleting from a queue
- program to remove a duplicate node from link list
- program to insert an element in the queue
- program to calculate the roots of fourth order alz...
- program to solve three variable linear equation
- simple program of railway reservation
- program to replace the substring with another subs...
- program to reverse each word of the string
- program to save the output as a bitmap image
- program of sparse matrix
- program to add, sub, mul ,and divide two complex n...
- program to find the area and circumference of circ...
- program to show the basics of inline function in oops
- program to print rectangle without using inbuilt f...
- program to print rectangular bowl without using in...
- program to print 'c graphic' in the lines without ...
- program to print menu without using inbuilt functions
- program of loading
- program of loading 2
- program of moving boundries
- program to print all the ascii values
- program to print a barcode
- program to print a line
- program to print a welcome screen?
- program to demonstrate setfillstyle
- program to select the different background colours
- program which demonstrate the text style
- program to demonstrate line style
- program to demonstrate the size of text
- program to print a baby
- program of loading 1
- program of loading 2
- program to print mickey mouse
- program to print a variable box
- program to print database using moving lights arou...
- program to print menu base in graphics
- program to print menu in very good graphic form
- program to enter the values in student database
- program to enter the values of data of form in box...
- program of main menu
- program which define the pixel of the cursor while...
- program to draw lines on the screen with the help ...
- program of moving 3dimensional rectangle box
- program to show menu with the cursor
- program which takes a number from keyboard and giv...
- program which draw rectangles on the screen with t...
- program of digital switches
- program to show the basics os screen saver
- program of text box
- project of editor(like notepad) in c++
- project of banking
- project of hostel management
- project of three dimensional calculator working wi...
- graphical project of students database
- project of shooting game
- Program to find the factorial of a large number
- Solved Placement papers of different IT companies
- there is a matrix N x N .Its elements consist of e...
- program to convert binary number into decimal numb...
- A string of charater is given.Find the highest occ...
- Two sentences are given and we were required to pr...
-
▼
September
(132)
program to enter the values of data of form in boxex and by pressing enter cursor goes to second box
Tuesday, September 1, 2009Posted by Upinder Singh Dhami at Tuesday, September 01, 2009
Labels: advance graphics
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment