#include <stdio.h>
#include <conio.h>
#include <graphics.h>
#include <alloc.h>
int SaveBMP16(char []);
typedef unsigned char byte;
typedef unsigned int word;
typedef unsigned long dword;
struct BMP
{
// BitMap File Header
/* 1 2 */ byte bfType[2]; // must always be set to 'BM' to declare that this is a .bmp file.
/* 3 4 */ dword bfSize; // specifies the size of the file in bytes.
/* 7 2 */ word bfReserved1; // must always set to zero.
/* 9 2 */ word bfReserved2; // must always be set to zero.
/* 11 4 */ dword bfOffset; // specifies the offset from the beginning of the file to the bitmap data.
// BitMap Image Header
/* 15 4 */ dword biSize; // specifies the size of the BitMap Header structure, in bytes.
/* 19 4 */ dword biWidth; // specifies the width of image, in pixels.
/* 23 4 */ dword biHeight; // specifies the height of image, in pixels.
/* 27 2 */ word biPlanes; // specifies the number of planes of the target device, must be set to zero.
/* 29 2 */ word biBitCount; // specifies the number of bits per pixel.
/* 31 4 */ dword biCompression; //Specifies the type of compression, usually set to zero (no compression).
/* 35 4 */ dword biSizeImage; //specifies the size of the image data, in bytes. If there is no compression, it is valid to set this member to zero.
/* 39 4 */ dword biXPelsPerMeter; //specifies the the horizontal pixels per meter on the designated targer device, usually set to zero.
/* 43 4 */ dword biYPelsPerMeter; //specifies the the vertical pixels per meter on the designated targer device, usually set to zero
/* 47 4 */ dword biClrUsed; //specifies the number of colors used in the bitmap, if set to zero the number of colors is calculated using the biBitCount member.
/* 51 4 */ dword biClrImportant; //specifies the number of color that are 'important' for the bitmap, if set to zero, all colors are important.
};
int SaveBMP16(char file[])
{
int i=0, j=0, r, g, b;
FILE *fp;
BMP *bmp;
bmp=(BMP *)malloc(54);
bmp->bfType[0]='B';
bmp->bfType[1]='M';
bmp->bfSize=153718;
bmp->bfReserved1=0;
bmp->bfReserved2=0;
bmp->bfOffset=118;
bmp->biSize=40;
bmp->biWidth=640;
bmp->biHeight=480;
bmp->biPlanes=1;
bmp->biBitCount=4;
bmp->biCompression=0;
bmp->biSizeImage=153600;
bmp->biXPelsPerMeter=0;
bmp->biYPelsPerMeter=0;
bmp->biClrUsed=0;
bmp->biClrImportant=0;
fp=fopen("c:\\testP.bmp", "wb");
if(fp == NULL)
{
printf("File can't be open");
getch();
return 1;
}
fwrite(bmp, 54, 1, fp);
fseek(fp, 54L, SEEK_SET);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(127, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(127, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(127, fp);
fputc(127, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(127, fp);
fputc(0x0, fp);
fputc(127, fp);
fputc(0x0, fp);
fputc(127, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(192, fp);
fputc(192, fp);
fputc(0x0, fp);
fputc(192, fp);
fputc(192, fp);
fputc(192, fp);
fputc(0x0, fp);
fputc(128, fp);
fputc(128, fp);
fputc(128, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(255, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(0x0, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(255, fp);
fputc(0x0, fp);
fputc(255, fp);
fputc(255, fp);
fputc(255, fp);
fputc(0x0, fp);
i=0;
j=479;
fseek(fp, 118, SEEK_SET);
while(j>=0)
{
i=0;
while(i<640)
{
fputc((getpixel(i, j)<<4) | getpixel(i+1, j), fp);
i+=2;
}
j--;
}
free(bmp);
fclose(fp);
return 0;
}
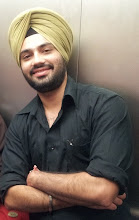
- Upinder Singh Dhami
- Bangalore, Karnataka, India
- Extending one hand to help someone has more value rather than joining two hands for prayer
Archives
-
▼
2009
(135)
-
▼
September
(132)
- program to print a random number
- program to remove the first and last occurance of ...
- program of selection sort
- program to reverse a string without using inbuilt ...
- program which show the basics of pointer
- program to count characters, words and lines in th...
- program to count vovels,digits,spaces,consonent an...
- program to sort a string
- program to find the number of substrings in a string
- program of password, where you write your password...
- program of dynamic merge
- program of dynamic sort
- program to toogle a string
- program which convert a float value to the string
- program to show the use of gotoxy
- program of merge sort
- calculate the occurance of a word in the string
- program to find the transpose of a matrix
- program to find the largest number in each row of ...
- program to find the sum of rows of the matrix
- program to find the sum of diagonal elements of th...
- program to sort the array using function
- program of seletion sort
- program to search a number present in number of ti...
- program to find the product of matrix
- program to insert the element in the array
- program for binary search of elements which are al...
- program to show basics of linklist
- program to count number of nodes in the link list
- program to count odd values of node in linklist
- program to search a node by value
- program to search a node by value and place a node...
- program to search a node by value and remove it
- program which domonstrate the use of tree
- program to remove alternate nodesin the link list
- program to reversea link list
- program to search a node by value and replace it w...
- program to remove duplicate nodes from the link list
- program to enter the name and email of students us...
- program to show inserting and deleting from a queue
- program to remove a duplicate node from link list
- program to insert an element in the queue
- program to calculate the roots of fourth order alz...
- program to solve three variable linear equation
- simple program of railway reservation
- program to replace the substring with another subs...
- program to reverse each word of the string
- program to save the output as a bitmap image
- program of sparse matrix
- program to add, sub, mul ,and divide two complex n...
- program to find the area and circumference of circ...
- program to show the basics of inline function in oops
- program to print rectangle without using inbuilt f...
- program to print rectangular bowl without using in...
- program to print 'c graphic' in the lines without ...
- program to print menu without using inbuilt functions
- program of loading
- program of loading 2
- program of moving boundries
- program to print all the ascii values
- program to print a barcode
- program to print a line
- program to print a welcome screen?
- program to demonstrate setfillstyle
- program to select the different background colours
- program which demonstrate the text style
- program to demonstrate line style
- program to demonstrate the size of text
- program to print a baby
- program of loading 1
- program of loading 2
- program to print mickey mouse
- program to print a variable box
- program to print database using moving lights arou...
- program to print menu base in graphics
- program to print menu in very good graphic form
- program to enter the values in student database
- program to enter the values of data of form in box...
- program of main menu
- program which define the pixel of the cursor while...
- program to draw lines on the screen with the help ...
- program of moving 3dimensional rectangle box
- program to show menu with the cursor
- program which takes a number from keyboard and giv...
- program which draw rectangles on the screen with t...
- program of digital switches
- program to show the basics os screen saver
- program of text box
- project of editor(like notepad) in c++
- project of banking
- project of hostel management
- project of three dimensional calculator working wi...
- graphical project of students database
- project of shooting game
- Program to find the factorial of a large number
- Solved Placement papers of different IT companies
- there is a matrix N x N .Its elements consist of e...
- program to convert binary number into decimal numb...
- A string of charater is given.Find the highest occ...
- Two sentences are given and we were required to pr...
-
▼
September
(132)
program to save the output as a bitmap image
Tuesday, September 1, 2009Posted by Upinder Singh Dhami at Tuesday, September 01, 2009
Labels: intermediate programs
Subscribe to:
Post Comments (Atom)
0 comments:
Post a Comment